Here is an example of a “Hello, World!” program in HTML:
<!DOCTYPE html>
<html>
<head>
<title>Hello, World!</title>
</head>
<body>
<h1>Hello, World!</h1>
</body>
</html>
To create an HTML file, you will need to use a plain text editor, such as Notepad on Windows or TextEdit on Mac. Save the file with an .html
extension, then open it in a web browser to see the result.
Here is an example of an HTML button with a tile style and a JavaScript function that is called when the button is clicked:
<!DOCTYPE html>
<html>
<head>
<style>
.tile-button {
display: block;
width: 100px;
height: 50px;
line-height: 50px;
text-align: center;
background-color: blue;
color: white;
font-size: 16px;
border-radius: 4px;
}
</style>
</head>
<body>
<button class="tile-button" onclick="alert('Button clicked!')">Click me</button>
</body>
</html>
The style
element in the head
section of the HTML defines the look of the button using CSS. The button
element in the body
section of the HTML creates the button and the onclick
attribute specifies the JavaScript function that should be called when the button is clicked. In this case, the function is alert('Button clicked!')
, which displays an alert box with the message “Button clicked!”.
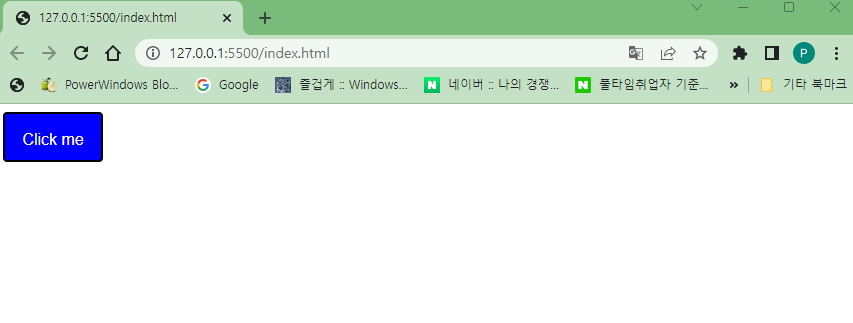
Here is an example of an HTML button with a tile style that displays the current date when clicked, using JavaScript:
<!DOCTYPE html>
<html>
<head>
<style>
.tile-button {
display: block;
width: 100px;
height: 50px;
line-height: 50px;
text-align: center;
background-color: blue;
color: white;
font-size: 16px;
border-radius: 4px;
}
</style>
</head>
<body>
<button class="tile-button" onclick="displayDate()">Click me</button>
<script>
function displayDate() {
var currentDate = new Date();
alert(currentDate);
}
</script>
</body>
</html>
The displayDate
function creates a new Date
object, which represents the current date and time, and displays it using the alert
function. When the button is clicked, the displayDate
function is called and the current date is displayed in an alert box.
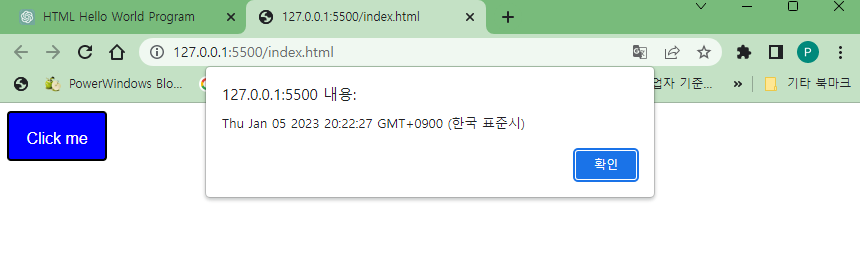
Leave a Reply